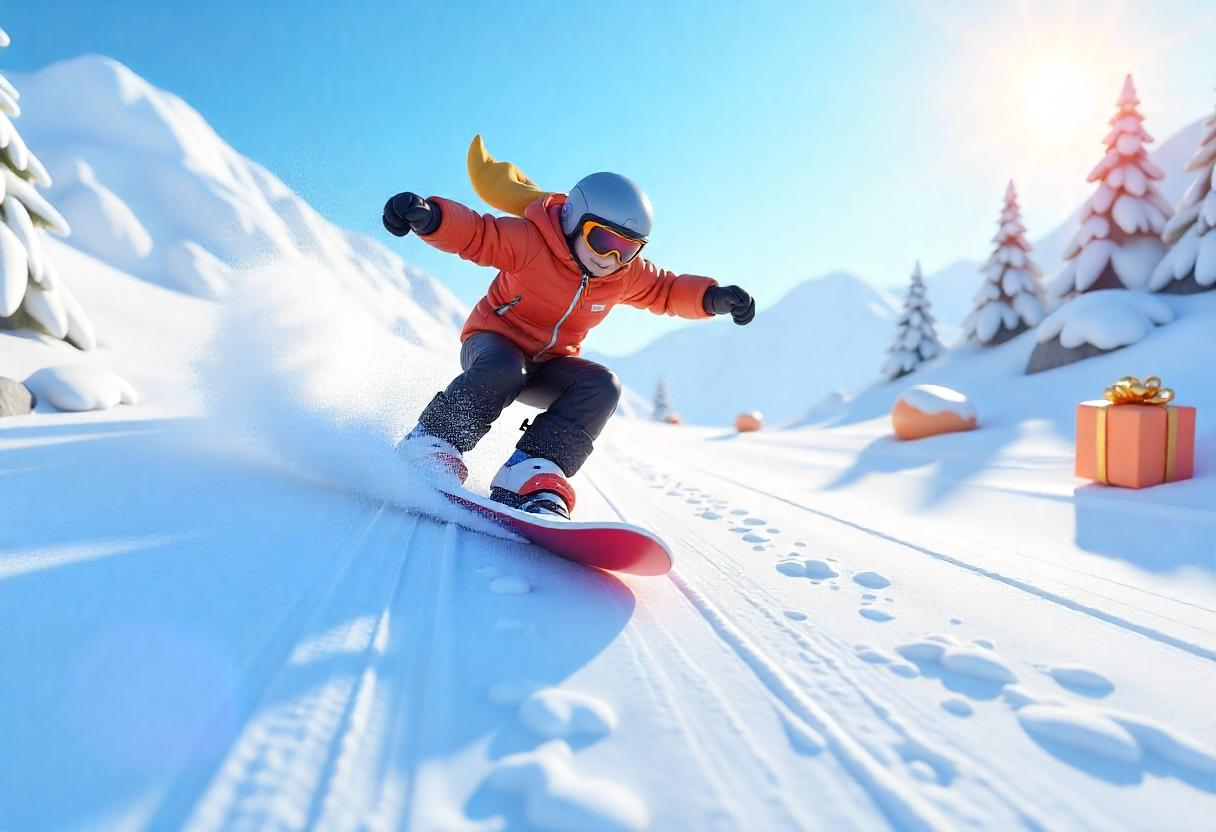
Table of Contents
- Introduction
- What is Snow Rider 3D & Snow Rider 3D GitHub?
- Setting Up Your Development Environment
- Install Unity
- Set Up GitHub
- Install a Code Editor
- Designing Your Snowboarding Game Concept
- Character Movement
- Obstacles & Collectibles
- Score System & Camera Angles
- Developing the Core Mechanics
- Creating a Snowboarder Character
- Generating Terrain & Snowy Slopes
- Adding Obstacles & Collectibles
- Implementing a Scoring System
- Enhancing the Gameplay Experience
- Camera Adjustments
- UI Elements (Score, Time, Health)
- Sound Effects & Background Music
- Testing & Optimization
- Debugging & Bug Fixing
- Performance Optimization
- Gathering User Feedback
- Uploading & Sharing on GitHub
- Creating a GitHub Repository
- Uploading Project Files
- Adding Documentation & Sharing
- Exploring Existing Snowboarding Game Projects on GitHub
- Contributing to Snowboarding Game Projects
- Forking & Cloning Repositories
- Making Changes & Submitting Pull Requests
- Conclusion
- FAQs
- Best Programming Language for 3D Games?
- Can I Modify Existing Snow Rider 3D GitHub Projects?
- How to Optimize Game Performance?
- How to Publish My Game After Development?
- Where to Find Free Game Assets?
Introduction
Snow Rider 3D is an exciting snowboarding game that has gained popularity for its smooth gameplay, challenging obstacles, and engaging mechanics. Players navigate snowy landscapes, dodging trees, rocks, and other obstacles while collecting gifts to boost their scores. The game’s simplicity, combined with its high replay value, makes it a fan favorite.
For game developers and enthusiasts, recreating a similar experience using GitHub is an excellent way to learn about 3D game development, programming, and game mechanics. This article provides a step-by-step guide on how to create a snowboarding game like Snow Rider 3D using Unity, GitHub, and essential programming concepts. Whether you’re a beginner or an experienced developer, this guide will help you understand the core aspects of game development, from setting up your environment to deploying your game.
What is Snow Rider 3D?
Snow Rider 3D is an online snowboarding game where players race down snowy mountains, avoiding obstacles while collecting items. The game features:
- Smooth Controls: Easy-to-use keyboard or touchscreen inputs for navigation.
- Challenging Gameplay: Increasing difficulty as the player progresses.
- Engaging Visuals: 3D graphics that create a realistic snowboarding experience.
- Reward System: Players earn points by collecting gifts and avoiding crashes.
The game’s addictive nature makes it appealing to casual players and competitive gamers alike.
For the best gaming experience, we recommend using a high-performance gaming laptop. Check out this top-rated gaming laptop on Amazon for smooth and lag-free gameplay: Click Here to View on Amazon
Step 1: Setting Up Your Development Environment
Before you begin developing your snowboarding game, you need to set up the right tools.
1. Install Unity
Unity is a powerful game engine that supports 3D game development. You can download it from the official Unity website. Make sure to select the latest LTS (Long-Term Support) version for the best stability.
2. Set Up GitHub
GitHub is essential for version control and collaboration. To integrate it into your project, follow these steps:
- Create a GitHub account at GitHub.com.
- Download Git and install it on your computer.
- Set up a new repository for your game project.
3. Install a Code Editor
While Unity has a built-in editor, Visual Studio Code or Visual Studio offers better debugging tools. Download and install one based on your preference.
Step 2: Designing Your Snowboarding Game Concept
Before jumping into coding, outline your game’s core features:
- Character Movement: The snowboarder should be able to move left, right, and jump.
- Obstacles: Add trees, rocks, and ramps to make the game challenging.
- Score System: Implement a point system where players earn rewards for collecting gifts.
- Game Environment: Design snowy mountains, slopes, and background scenery.
- Camera Angles: Decide on a third-person or top-down view.
Step 3: Developing the Core Mechanics
1. Create a Snowboarder Character
- Use Unity’s 3D models or import a character from online asset stores.
- Apply Rigidbody physics to simulate realistic movement.
- Attach a script to control movement:
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float speed = 10f;
public float turnSpeed = 5f;
void Update()
{
float move = Input.GetAxis("Vertical") * speed * Time.deltaTime;
float turn = Input.GetAxis("Horizontal") * turnSpeed * Time.deltaTime;
transform.Translate(0, 0, move);
transform.Rotate(0, turn, 0);
}
}
2. Generate the Terrain
- Use Unity’s Terrain tool to create mountains and snow-covered paths.
- Apply snow textures from free asset libraries.
3. Add Obstacles and Collectibles
- Use Prefab system to create reusable obstacles.
- Implement a script for collision detection:
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("Obstacle"))
{
Debug.Log("Game Over!");
}
}
4. Implement a Scoring System
- Add a script to count points when the player collects gifts.
public int score = 0;
void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("Gift"))
{
score += 10;
Destroy(other.gameObject);
}
}
Step 4: Enhancing the Gameplay Experience
1. Camera Adjustments
- Use Cinemachine in Unity for smooth camera tracking.
- Set the camera to follow the player dynamically.
2. UI Elements
- Display score, time, and health using Unity’s UI Canvas.
3. Sound Effects & Music
- Add background music and sound effects for jumps and crashes.
Step 5: Testing & Optimization
Before publishing your game, ensure:
- Bug Fixing: Test for movement glitches, collision issues, and scoring errors.
- Performance Optimization: Reduce unnecessary assets to enhance frame rates.
- User Feedback: Ask friends or fellow developers to test your game.
Step 6: Uploading & Sharing on GitHub
1. Create a GitHub Repository
Go to GitHub.com and create a new repository.
2. Upload Project Files
Use Git commands to push your project:
git init
git add .
git commit -m "Initial commit"
git branch -M main
git remote add origin
git push -u origin main
3. Share Your Work
- Add a README.md file with installation instructions.
- Encourage contributions from other developers.
Exploring Existing Snowboarding Game Projects on GitHub
For reference, check out these GitHub projects:
- Snowboarding by tahsinsoha – A Unity-based snowboarding game with collectibles and scoring.
- Snowboarder by mrbid – A simple yet engaging snowboarding experience.
Contributing to Snowboarding Game Projects
Want to contribute to Snow Rider 3D or similar GitHub projects? Follow these steps:
- To fork the repository – simply click the “Fork” button on GitHub.
- Clone the Repository – Use
git clone
to download the files. - Make modifications – Introduce new features or resolve issues.
- Submit a Pull Request – Request the owner to merge your changes.
Conclusion
Creating a snowboarding game like Snow Rider 3D using GitHub is an exciting journey that blends creativity with technical skills. By following this guide, you can develop an immersive game, share it with the community, and even contribute to existing projects. Whether you’re a beginner or an experienced developer, this project is a great way to enhance your game development skills.
Happy coding, and may your snowboarding adventure be a smooth ride! 🚀
FAQs
1. What programming language should I use for a 3D game?
C# is the best choice, especially with Unity, due to its powerful scripting capabilities.
2. Can I modify existing Snow Rider 3D GitHub projects?
Yes, but check the license to ensure modifications and distributions are allowed.
3. How can I optimize my game for better performance?
Reduce unnecessary assets, optimize textures, and use Object Pooling for repeated elements.
4. Can I publish my snowboarding game after development?
Yes! You can release it on platforms like itch.io, Google Play, or the App Store.
5. Where can I find free assets for my game?
Check Unity Asset Store, OpenGameArt, and Free3D for free 3D models and textures.
Please don’t forget to leave a review.
Do you want a this game will create for you contact us We available every time for you